Signing requests for key operations
Why do you need to do this?
This is done to add an extra layer of security to actions that impact the outflow of funds from the Volt Account.
How do you do this using the API?
We use JWS (JSON Web Signature) as the signature method for each request to authenticate it using a second factor in addition to API credentials.
What happens if you don't sign each API request?
Your request won’t be processed for payment automatically, but will instead appear in a queue for processing in Fuzebox. You will need to login to Fuzebox and authorise the request using a passkey stored on your computer or mobile device. This can be done in bulk or for each individual payment.
Where can you test this?
You can test the signing on sandbox and production. However, we recommend testing it particularly on production before activating it for your shoppers.
Integration steps
First, you’ll need to set up the trusted signing keys. Second, for each request, create a JWT (JSON Web Token) which includes the signature and include that in the request header.
1) Setting up a key for signing
- Create private / public key pair
- Store the private key in a secure manner
- Share public key with Volt and get your assigned public key id
2) Signing the requests
Once you have the private key and the public key id you can proceed with signing the requests.
Create key pair
Technical specifications
- We support public keys in the following formats: PKCS1, PKCS8 and x509.
- The public key length should be between 2048 and 4096 bytes.
How to generate your keys
It is recommended that you will use your own PKI infrastructure preferably based on a hardware security module (HSM) to generate a key pair for this use case as this is industry best practice.
However, as not all companies are able to manage such solutions below recommendations were prepared to help with that manner.
The first option is to use a key creation functionality in Fuzebox. To do this go to Configuration > Customers> API Access> Signature Keys> Generate a key pair . Your public key will be automatically saved and you will receive a private key and public key id in a popup message. Please bear in mind that Volt is not saving on its side this private key.
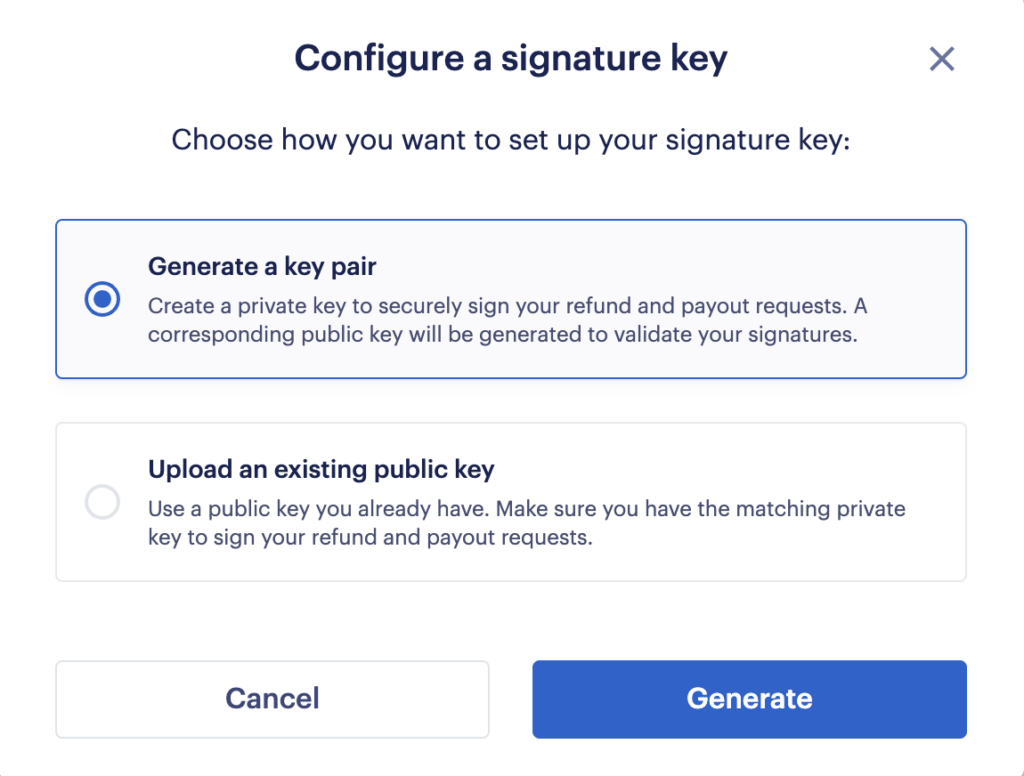
You can also generate your private / public key pair using a terminal which supports the openssl command, and by following the details listed above.
If you generated the key pair outside of Fuzebox (e.g. in Terminal)” please upload your public key to Fuzebox under Configuration > Customers> API Access>Signature Keys> Upload an existing public key.
Once you upload the public key there, you will get a key ID, a UUID which you’ll need to use when generating your signed token on each refund or payout request, so please keep this ID safe.
Example of an ID is 901659f9-c0fd-4d2e-82b8-a55f51f80d73
.
You should never share your private key with Volt, or with any third party. If you believe your private key may be compromised, you should immediately inform Volt, generate new a key pair and send us the new public key.
Create a JWT token for each request
To sign each request, you will need to provide a JWT token in the header of that request. To generate a signed JWT token, you’ll need your private key and the ID of your public key, plus the payload (the JSON body) of the POST request for your Refund or Payout.
Creating your JWT token
You need to create additional two parts for your JWT token, the header and the signature supporting actual payload with data. The header tells us how to process the authentication of payload data and compare results with the signature provided to validate integrity and authenticity of the whole JWT token.
The token header segment
The header should be created first as a JSON object as below, where
alg
– represents the algorithm used to encode/decode the token, in this case it’sRS256
(the Rsa+Sha256 algorithm)typ
– should be “JWT
“kid
– theid
of the public key that we supplied you, which will be in UUID form
{
"alg": "RS256",
"typ": "JWT",
"kid": "ce161c49-4373-4b07-82fa-217998f6b3e8"
}
Encoding the header
Once you’ve created the JSON object as per the example above, you should remove any extra spaces or line breaks, then encode it using the base64UrlEncoded algorithm, as per the example below.
tokenHeader = '{"alg":"RS256","typ":"JWT","kid":"ce161c49-4373-4b07-82fa-217998f6b3e8"}'
encodedHeader = base64UrlEncode(tokenHeader)
… which should then look like the example below.
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6ImNlMTYxYzQ5LTQzNzMtNGIwNy04MmZhLTIxNzk5OGY2YjNlOCJ9
The signature segment
To create the signature segment, we need to use the algorithm that is specified in the header and the private key. This is where you’ll need a copy of the payload you’re sending in the POST request, because that’s what you’re actually signing here.
In this case we’re using the RSA+SHA256 algorithm and would use the sample code below, utilising the already encoded header plus a base64UrlEncoded version of the JSON payload you will be sending in the refund or payout request. You should not change the payload after generating the signature as this will invalidate the signed token.
Remove whitespace to improve validation
Please remove any line breaks or extra spaces in the request payload before using the base64UrlEncode function, this will ensure that your signature matches the way we’ll validate it. For example, in the case of refunds, you should base64UrlEncode('{"amount":1,"externalReference":"my-external-reference"}')
tokenHeader = '{"alg": "RS256","typ":"JWT","kid":"ce161c49-4373-4b07-82fa-217998f6b3e8"}'
requestPayload = '{"amount":1,"externalReference":"my-external-reference"}'
signature = RSASHA256( base64UrlEncode(tokenHeader) + "." + base64UrlEncode(requestPayload), privateKey )
If all is good you should get a signature which looks like this…
oUjLLtQigBniTiYswfE0JAjMiYXtIlNtVi1Lr1jqBx103vXgVtEdWApUMpG3wze3qVXD_APA2Sk8oLV4DGeBb5pN7yRGeCdxoV3IsikCVs6rn2Q2Jat-bReQMX39F7-Rpn7RznjHUsyWWcNbDKy1wRFcEnDJBVdb_1lKdFBPWaKMkB1Yd8t8X2va6mq7pJXPAMS36Gwc37vULZvdw4D-49r8mcbEGnNXwkcuZ08hMk4UsmM0kxLeNcrVD3wZtuU0N43u1trlPnuX9RDOOh9Gz0fEH1fwxdveAZaMOOWr7IPHBeV8nZXHxt1lpwJ-dpAsSDMvCFhr-MHuOQDDdqsfhQ
Build your JWT token
To build the final JWT token to sign your request, take the encoded header and join it to the signature as follows.
JWT = base64UrlEncode(header) + ".." + signature // Note the ".."
Important note
Please note the double dot ".."
is important when building the JWT token. Validation will fail if you miss one of the dots.
So, using the examples given in this page, your final token should look like the example below:
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6ImNlMTYxYzQ5LTQzNzMtNGIwNy04MmZhLTIxNzk5OGY2YjNlOCJ9..oUjLLtQigBniTiYswfE0JAjMiYXtIlNtVi1Lr1jqBx103vXgVtEdWApUMpG3wze3qVXD_APA2Sk8oLV4DGeBb5pN7yRGeCdxoV3IsikCVs6rn2Q2Jat-bReQMX39F7-Rpn7RznjHUsyWWcNbDKy1wRFcEnDJBVdb_1lKdFBPWaKMkB1Yd8t8X2va6mq7pJXPAMS36Gwc37vULZvdw4D-49r8mcbEGnNXwkcuZ08hMk4UsmM0kxLeNcrVD3wZtuU0N43u1trlPnuX9RDOOh9Gz0fEH1fwxdveAZaMOOWr7IPHBeV8nZXHxt1lpwJ-dpAsSDMvCFhr-MHuOQDDdqsfhQ
Sign your request
Now you have your request payload and a JWT token which signs that payload, all you need to do is add a JWS header to each request, which contains the JWT token you created.
Header name : X-JWS-Signature
X-JWS-Signature: eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6ImNlMTYxYzQ5LTQzNzMtNGIwNy04MmZhLTIxNzk5OGY2YjNlOCJ9..oUjLLtQigBniTiYswfE0JAjMiYXtIlNtVi1Lr1jqBx103vXgVtEdWApUMpG3wze3qVXD_APA2Sk8oLV4DGeBb5pN7yRGeCdxoV3IsikCVs6rn2Q2Jat-bReQMX39F7-Rpn7RznjHUsyWWcNbDKy1wRFcEnDJBVdb_1lKdFBPWaKMkB1Yd8t8X2va6mq7pJXPAMS36Gwc37vULZvdw4D-49r8mcbEGnNXwkcuZ08hMk4UsmM0kxLeNcrVD3wZtuU0N43u1trlPnuX9RDOOh9Gz0fEH1fwxdveAZaMOOWr7IPHBeV8nZXHxt1lpwJ-dpAsSDMvCFhr-MHuOQDDdqsfhQ
Test it with Postman
We have a test script in our Postman collection. It is part of the “Scripts > Pre-req”.
For testing you need
- Private key for sandbox (not your production private key)
- Public key id (kid) from Volt
Simple examples of code
- Java
- PHP
- JavaScript (NodeJS)
- Elixir
package com.example;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.security.KeyFactory;
import java.security.Security;
import java.security.interfaces.RSAPrivateKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.util.Base64;
import java.util.HashMap;
import java.util.Map;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class Refund {
public static void main(String[] args) throws Exception {
String baseUrl = System.getenv("BASE_URL");
String authToken = System.getenv("AUTH_TOKEN");
String kid = System.getenv("KID");
// Payload
Map<String, Object> payload = new HashMap<>();
payload.put("externalReference", "external_reference");
payload.put("amount", "100");
Output.print("payload:");
Output.print(payload.toString());
String paymentId = "83c17110-8d3c-4d96-9095-400b8f65c625";
// Load private key
RSAPrivateKey privateKey = getPrivateKey("./../private.txt");
// Create JWT
Algorithm algorithm = Algorithm.RSA256(null, privateKey);
String jwtString = JWT.create()
.withHeader(Map.of("alg", "RS256", "kid", kid, "typ", "JWT"))
.withPayload(payload)
.sign(algorithm);
// Prepare the parsed JWT string
String[] parts = jwtString.split("\\.");
String parsedJwtString = parts[0] + ".." + parts[2];
Output.print("JWT:");
Output.print(parsedJwtString);
// Convert payload to JSON
ObjectMapper objectMapper = new ObjectMapper();
String requestBody = objectMapper.writeValueAsString(payload);
Output.print("Request body:");
Output.print(requestBody);
// Set up the request
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create(baseUrl + "/payments/" + paymentId + "/request-refund"))
.header("Content-Type", "application/json")
.header("X-JWS-Signature", parsedJwtString)
.header("Authorization", "Bearer " + authToken)
.POST(HttpRequest.BodyPublishers.ofString(requestBody))
.build();
// Send the request and handle the response
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
Output.print("Response:");
Output.print(response.body());
}
private static RSAPrivateKey getPrivateKey(String filename) throws Exception {
String privateKeyPEM = new String(Files.readAllBytes(Paths.get(filename)))
.replace("-----BEGIN PRIVATE KEY-----", "")
.replace("-----END PRIVATE KEY-----", "")
.replaceAll("\\s", "");
byte[] encoded = Base64.getDecoder().decode(privateKeyPEM);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(encoded);
return (RSAPrivateKey) keyFactory.generatePrivate(keySpec);
}
}
package com.example;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.security.KeyFactory;
import java.security.Security;
import java.security.interfaces.RSAPrivateKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.util.Base64;
import java.util.HashMap;
import java.util.Map;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class Payout {
public static void main(String[] args) throws Exception {
String baseUrl = System.getenv("BASE_URL");
String authToken = System.getenv("AUTH_TOKEN");
String kid = System.getenv("KID");
// Payload
Map<String, Object> payload = new HashMap<>();
payload.put("amount", 1);
payload.put("currency", "EUR");
payload.put("paymentTitle", "testEUR");
Map<String, String> beneficiary = new HashMap<>();
beneficiary.put("name", "test account");
beneficiary.put("iban", "DE07500105176551562526");
beneficiary.put("swiftBic", "BIGBPLPW");
payload.put("beneficiary", beneficiary);
// Load private key
RSAPrivateKey privateKey = getPrivateKey("./../private.txt");
// Create JWT
Algorithm algorithm = Algorithm.RSA256(null, privateKey);
String jwtString = JWT.create()
.withHeader(Map.of("alg", "RS256", "kid", kid, "typ", "JWT"))
.withPayload(payload)
.sign(algorithm);
Output.print("payload:");
Output.print(payload.toString());
// Prepare the parsed JWT string
String[] parts = jwtString.split("\\.");
String parsedJwtString = parts[0] + ".." + parts[2];
Output.print("JWT:");
Output.print(parsedJwtString);
// Convert payload to JSON
ObjectMapper objectMapper = new ObjectMapper();
String requestBody = objectMapper.writeValueAsString(payload);
Output.print("Request body:");
Output.print(requestBody);
// Set up the request
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create(baseUrl + "/payouts"))
.header("Content-Type", "application/json")
.header("X-JWS-Signature", parsedJwtString)
.header("Authorization", "Bearer " + authToken)
.POST(HttpRequest.BodyPublishers.ofString(requestBody))
.build();
// Send the request and handle the response
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
Output.print("Response:");
Output.print(response.body());
}
private static RSAPrivateKey getPrivateKey(String filename) throws Exception {
String key = new String(Files.readAllBytes(Paths.get(filename)));
String privateKeyPEM = key
.replace("-----BEGIN PRIVATE KEY-----", "")
.replace("-----END PRIVATE KEY-----", "")
.replaceAll("\\s", "");
byte[] encoded = Base64.getDecoder().decode(privateKeyPEM);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(encoded);
return (RSAPrivateKey) keyFactory.generatePrivate(keySpec);
}
}
/**
* This example uses:
* - vlucas/phpdotenv
* - firebase/php-jwt
*/
<?php
require 'vendor/autoload.php';
use Firebase\JWT\JWT;
use Dotenv\Dotenv;
// Load environment variables
$dotenv = Dotenv::createImmutable(__DIR__.'/../');
$dotenv->load();
$baseUrl = getenv('BASE_URL');
$authToken = getenv('AUTH_TOKEN');
$kid = getenv('KID');
// Payload and header
$paymentId = '83c17110-8d3c-4d96-9095-400b8f65c625';
$payload = ["externalReference"=>"external_reference","amount"=>100];
$header = [
"alg" => "RS256",
"kid" => $kid,
"typ" => "JWT"
];
// Read private key from file
$privateKey = file_get_contents(__DIR__ . '/../private.txt');
// Generate the JWT string
$jwtString = JWT::encode($payload, $privateKey, 'RS256', null, $header);
// Modify the JWT string for X-JWS-Signature header format
$parts = explode('.', $jwtString);
$parsedJwtString = $parts[0] . '..' . $parts[2];
// Prepare headers
$headers = [
'Content-Type' => 'application/json',
'X-JWS-Signature' => $parsedJwtString,
'Authorization' => 'Bearer ' . $authToken
];
// Prepare body
$body = json_encode($payload);
// Output the parsed JWT string and body for verification
echo "JWT: $parsedJwtString\n";
echo "Body: $body\n";
/**
* This example uses dependencies:
* {
* "dependencies": {
* "fs-extra": "^11.2.0",
* "jws": "^4.0.0"
* }
* }
*/
const jws = require('jws');
const fs = require('fs-extra');
const baseUrl = process.env.BASE_URL;
const authToken = process.env.AUTH_TOKEN;
const paymentId = '7dade9eb-24d5-46d0-98bb-6248c995b276';
//payout
const payload = {
"externalReference": "external_reference",
"amount":100
};
// public key id
const kid = process.env.KID;
const header = {
"alg": "RS256",
"kid": kid,
"typ": "JWT"
};
//private key to sign, public key generated form this key should be stored by fuzebox
const privateKey = fs.readFileSync('./../private.txt').toString()
const jwtString = jws.sign({
header: header,
payload: payload,
privateKey: privateKey,
})
const parts = jwtString.split('.');
const parsedJwtString = parts[0] + '..' + parts[2];
console.log(parsedJwtString);
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("X-JWS-Signature", parsedJwtString);
myHeaders.append("Authorization", `Bearer ${authToken}`);
const body = JSON.stringify(payload);
console.log(body);
const response = fetch(`${baseUrl}/payments/${paymentId}/request-refund`, {
method: 'POST',
headers: myHeaders,
body: body,
}).then(response => {
response.json().then(response => {
console.log(response);
});
});
/**
* This example uses dependencies:
* {
* "dependencies": {
* "fs-extra": "^11.2.0",
* "jws": "^4.0.0"
* }
* }
*/
const jws = require('jws');
const fs = require('fs-extra');
const baseUrl = process.env.BASE_URL;
const authToken = process.env.AUTH_TOKEN;
//payout
const payload = {
"amount": 1,
"currency": "EUR",
"paymentTitle": "testEUR",
"beneficiary": {
"name": "test account",
"iban": "DE07500105176551562526",
"swiftBic": "BIGBPLPW"
}
};
// public key id
const kid = process.env.KID;
const header = {
"alg": "RS256",
"kid": kid,
"typ": "JWT"
};
//private key to sign, public key generated form this key should be stored by fuzebox
const privateKey = fs.readFileSync('./../private.txt').toString()
const jwtString = jws.sign({
header: header,
payload: payload,
privateKey: privateKey,
})
const parts = jwtString.split('.');
const parsedJwtString = parts[0] + '..' + parts[2];
console.log(parsedJwtString);
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("X-JWS-Signature", parsedJwtString);
myHeaders.append("Authorization", `Bearer ${authToken}`);
const body = JSON.stringify(payload);
console.log(body);
const response = fetch(`${baseUrl}/payouts`, {
method: 'POST',
headers: myHeaders,
body: body,
}).then(response => {
response.json().then(response => {
console.log(response);
});
});
# payout.exs
Mix.install([
{:jose, "~> 1.11"},
{:finch, "~> 0.14"},
{:jason, "~> 1.4"}
])
# Start Finch and register it as :my_finch
Finch.start_link(name: :my_finch)
# Load environment variables
base_url = System.get_env("BASE_URL")
auth_token = System.get_env("AUTH_TOKEN")
kid = System.get_env("KID")
private_key_path = "./../private.txt"
# Define payload
payload = %{
"amount" => 100,
"currency" => "EUR",
"paymentTitle" => "40eb4e15-945b-458c-b9d5-39ff89dac19c",
"beneficiary" => %{
"name" => "VADIM LANDA",
"iban" => "EE062200221017166033"
}
}
# Define header
header = %{
"alg" => "RS256",
"kid" => kid,
"typ" => "JWT"
}
# Read private key
private_key = File.read!(private_key_path)
# Create JWS token
jws = JOSE.JWK.from_pem(private_key)
jwt = JOSE.JWS.sign(jws, Jason.encode!(payload), %{ "alg" => "RS256", "kid" => kid })
|> JOSE.JWS.compact
|> elem(1)
# Parse the JWT
[header_part, _payload_part, signature_part] = String.split(jwt, ".")
parsed_jwt = "#{header_part}..#{signature_part}"
IO.puts("Parsed JWT: #{parsed_jwt}")
# Define headers
headers = [
{"Content-Type", "application/json"},
{"X-JWS-Signature", parsed_jwt},
{"Authorization", "Bearer #{auth_token}"}
]
# Set a 30-second timeout
options = [receive_timeout: 30_000]
# Send the request
Finch.build(:post, "#{base_url}/payouts", headers, Jason.encode!(payload))
|> Finch.request(:my_finch, options)
|> then(fn
{:ok, response} -> IO.inspect(response.body, label: "Response Body")
{:error, error} -> IO.inspect(error, label: "Error")
end)
# Dockerfile
FROM elixir:1.14
# Install dependencies
RUN apt-get update && apt-get install -y \
build-essential \
&& rm -rf /var/lib/apt/lists/*
# Install Finch
RUN mix local.hex --force && mix local.rebar --force
RUN mix archive.install hex phx_new --force
# Create app directory
WORKDIR /app
## Copy application files
#COPY payout.exs ./
#COPY private.txt ./
#
## Set environment variables
#ENV BASE_URL="your_base_url"
#ENV AUTH_TOKEN="your_auth_token"
#ENV KID="your_kid"
#
## Run the script
#CMD ["elixir", "payout.exs"]